First, we create a simple Java Class which will serve as the bean. Essentially, this class contains the business logic for our use case:
- It can return a list of available time zones
- It can return the current time depending on a specific time zone
- The time zone to use can be set through a setter method
package com.example.adf.view.bean; package com.example.adf.view.bean; import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.ArrayList; import java.util.List; import java.util.TimeZone; public class DateBean { private String timeZone = "Europe/Berlin"; /** * @return A list of available time zones. */ public List<String> getTimezones() { List<String> result = new ArrayList<>(); result.add("Europe/Berlin"); result.add("Asia/Calcutta"); result.add("US/Eastern"); result.add("America/Los_Angeles"); return result; } /** * @param timeZone The time zone to be used when returning the current time. */ public void setTimezone(String timeZone) { this.timeZone = timeZone; } /** * @return The current time in the selected time zone, in the format HH:mm:ss */ public String getCurrentTime() { DateFormat df = new SimpleDateFormat("HH:mm:ss"); TimeZone tz = TimeZone.getTimeZone(timeZone); df.setTimeZone(tz); return df.format(System.currentTimeMillis()); } }
Then, all we need to do to create the Data Control for this bean is to select the Java Class in the project navigator and choose “Create Data Control” from the context menu:
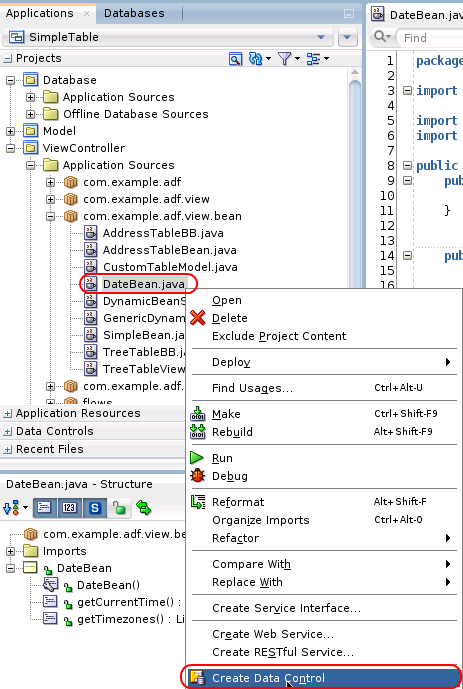
JDeveloper will query the name for the data control (which is the java class name by default, we are adding the suffix “DataControl” in order to make clear that this is a Data Control):
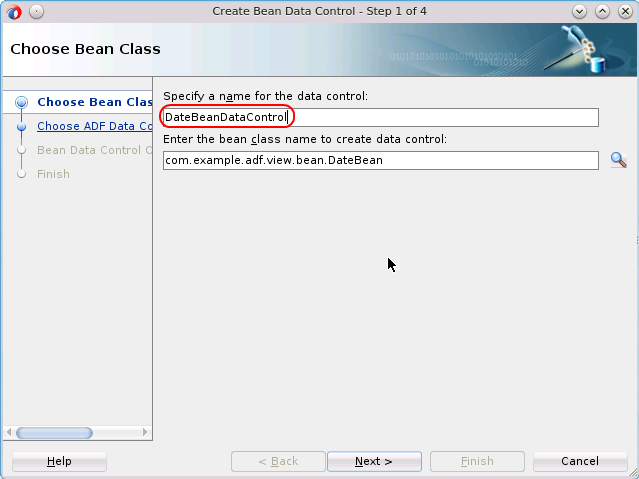
On the next two screens, JDeveloper allows to set some more details for the Data Control – we will keep the defaults for now:
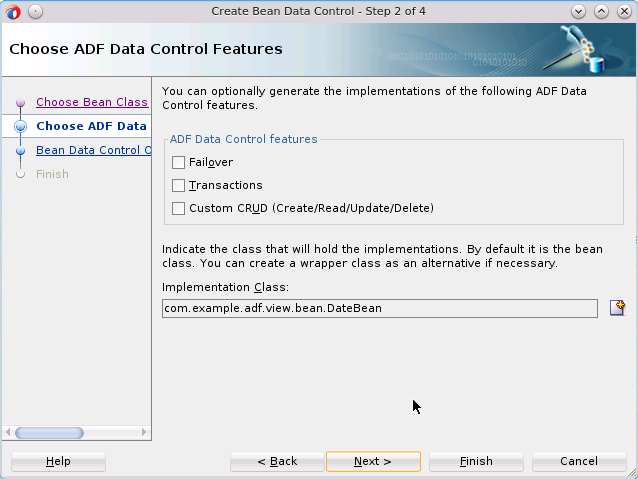
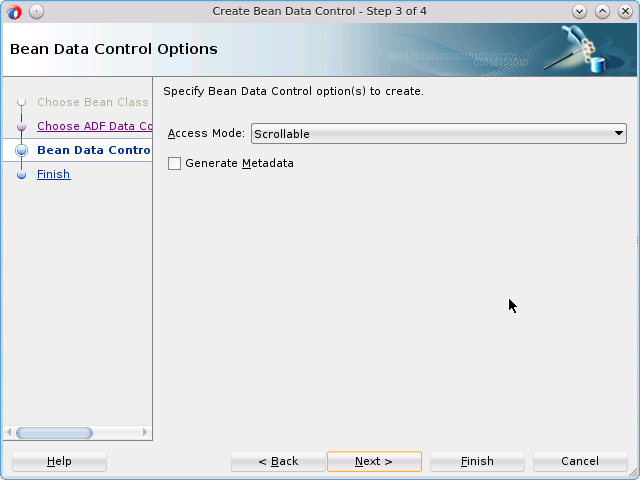
Press “Finish” to create the Data Control. After pressing the “Refresh” button on the Data Control palette, we can see that the new Data Control has been created:
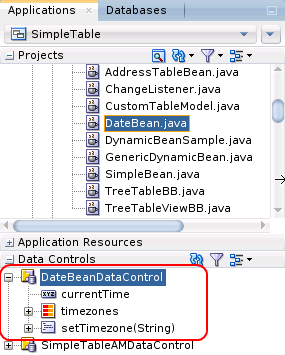
There is one attribute (currentTime) and one collection (timezones) available – each getter in the Java Class is exposed as either an attribute or a collection, depending on its return type. setTimeZone is a public method and exposed as a method call.
The next page shows how to implement the user interface using the data control which we just created: Implementing the UI